Transmitting data to the Philips P2000C#
In the previous blogpost it was shown how data can be sent from the P2000C to a modern computer. In this post, we will look into how we can reverse the process and transmit data from a modern computer to the P2000C.
Revised setup#
Since the previous blogpost, I also replaced my improvised set-up for a somewhat more professional solution as can be seen in the image below.
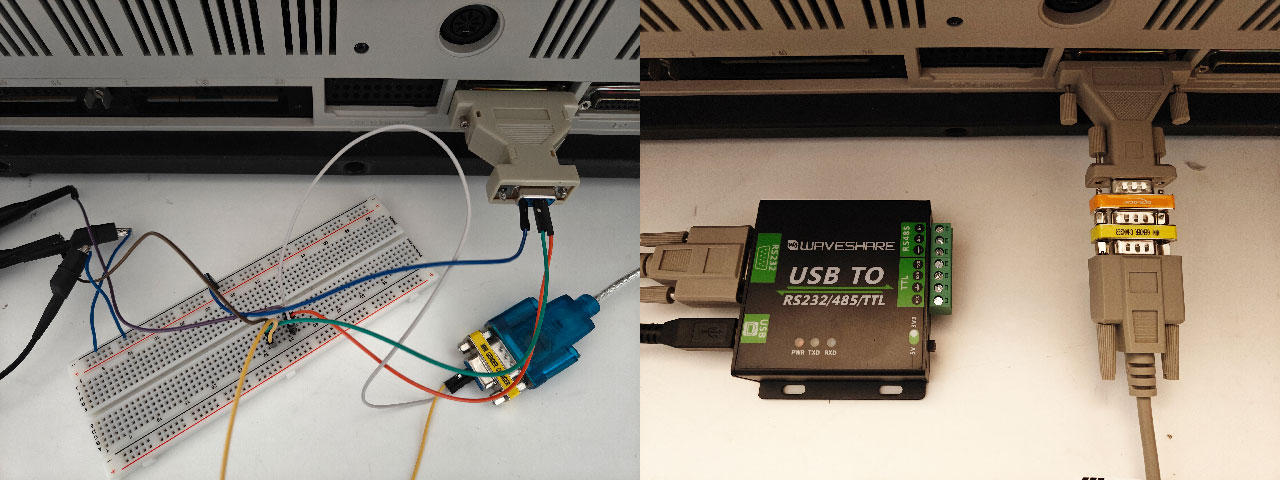
Previously, I have been using a cheap cable acquired from a popular Chinese website utilizing a CH340G chip which I hooked up using a number of jumper wires and a breadboard. In my new setup, I use a Waveshare RS232/TTL interface sporting a FT232RL chip. The breadboard and jumper wires are replaced with a proper data cable which is hooked up to the P2000C via a null-modem adapter (the orange block).
Receiving data#
Receiving data on the P2000C is done using yet another interrupt routine,
specifically 14,2
. This interrupt call will try to retrieve a byte from
the serial port into register AL
. If nothing is received after 20
seconds, the most significant bit (MSB) in AH
is set to 1
.
Taking this information into account, it is fairly straightforward to construct
a simple 8088 assembly routine that aims to consume bytes over the serial port
until a timeout is raised. The corresponding 8088-assembly code is provided in
the listing below.
mov ah,2
int 14h ; retrieve byte from serial port
and ah,80h ; check if MSB in AH is set
jnz 111 ; if so, timeout is raised, exit routine
mov dl,al
mov ah,2
int 21h ; print byte in DL to screen
jmp 100h ; try to consume another byte
int 20h ; exit program
On the modern computer, we of course need to send bytes to the P2000C. For that, I wrote a very small Python script that uses the PySerial library for the serial communication. In the script, a serial port is opened (using the right protocol parameters) and the string “Hello World!” is transmitted. Note that the string has to be encoded to ASCII before it can be sent over.
import serial
def main():
ser = serial.Serial('COM5', 1200, timeout=10)
if not ser.isOpen():
ser.open()
ser.write('Hello World!'.encode('ascii'))
ser.close()
if __name__ == '__main__':
main()
As there is no handshaking protocol whatsoever, the order in which the programs are executed matters. Since the P2000C uses a blocking call for retrieving bytes over the serial port, it is best to first run the P2000C program. Once started, we basically have 20 seconds to run the Python script before the timeout is generated, which is of course more than enough time. As soon as the modern computer transmits bytes, they are displayed on the screen of the P2000C. The result of the two programs can be seen in the image below.
Note
Note that DEBUG.COM uses hexadecimal notation everywhere, thus the listing shown on the screen differs slightly from that shown above. For the latter, I use the suffix h to indicate that the number of written in hexadecimal notation.
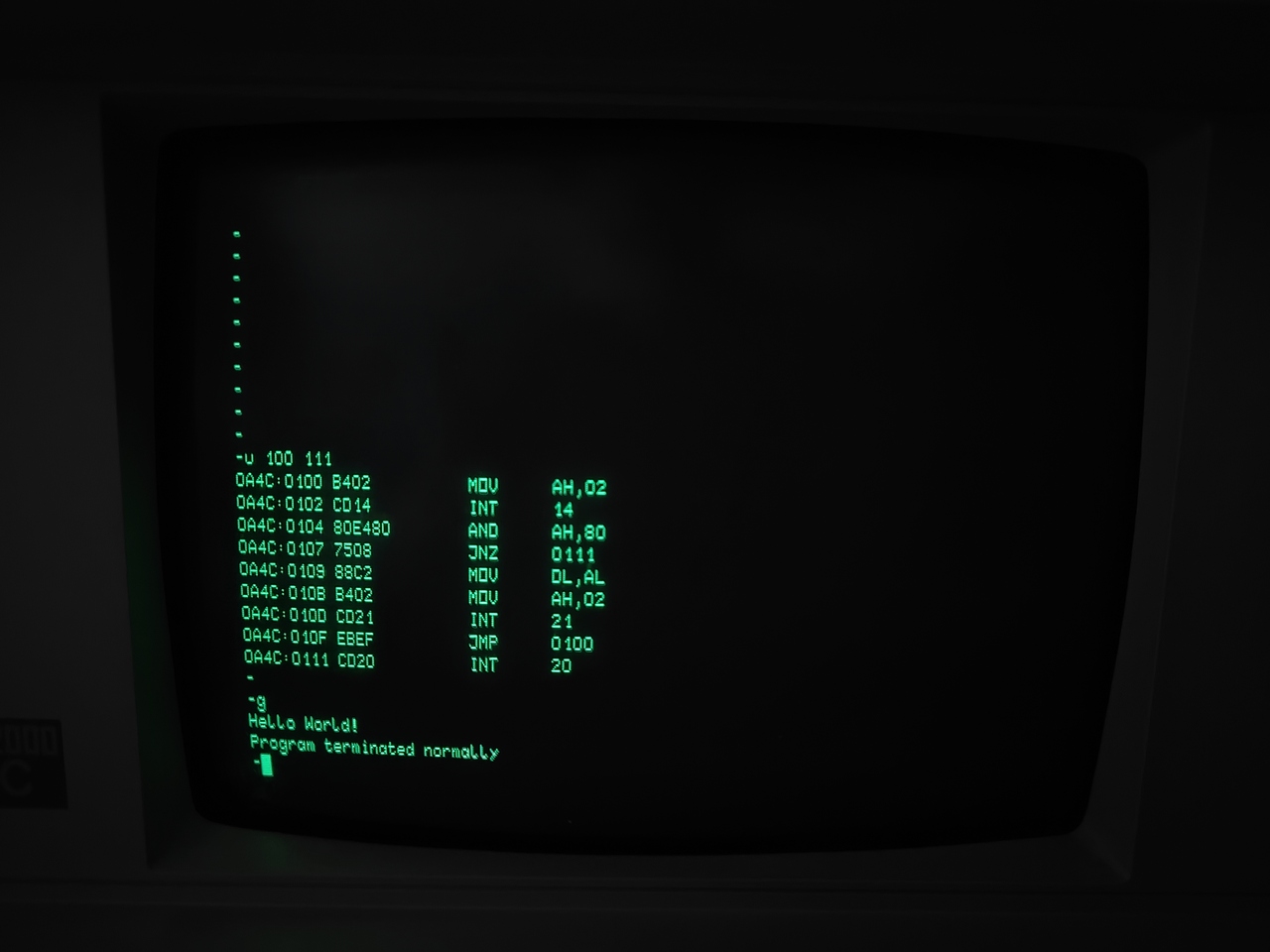
Next steps#
After successfully implementing both data transmission and reception, we are now well-equipped to develop a transfer program. The primary function of this program will be to transmit a file from a modern computer to the P2000C, where it will be automatically saved onto a floppy disk.
The creation of such a transfer program presents several significant advantages. Firstly, it enables us to download MS-DOS programs from the internet and transfer them to the P2000C. Additionally, this setup allows us to use the modern computer as a development environment, deploying the resulting programs to the P2000C, rather than developing everything directly on the P2000C.
While the DEBUG.COM tool is useful, writing programs with inline assembly can be quite cumbersome. More advanced assemblers, which support labels and macros, greatly simplify the process. Moreover, modern computers also provide the benefit of using compilers, further enhancing development efficiency.